Rangefinder
This script uses raycasting to measure distances:
-- This is a comment. Any text after -- is ignored by Lua.
--
-- It is considered good practice to document your code. Try to explain why
-- things happen. The code should describe how. We will deviate from this
-- recommendation here in order to provide a more thorough explanation.
--
-- Create a new behaviour called Rangefinder. Use behaviour() instead of
-- class() to enable hot-reloading.
behaviour("Rangefinder")
-- This function is called once every frame.
function Rangefinder:Update()
-- Create a ray originating from the object's origin position, pointing
-- in the object's forwards direction. Forwards is along the Z-axis
-- (the blue axis in the Unity Editor.) The "object" refereed to here
-- is the Unity GameObject who's ScriptedBehaviour component is
-- executing this Ravenscript.
local ray = Ray(self.transform.position, self.transform.forward)
-- Detect intersections with objects on the ProjectileHit layer such as
-- enemies, vehicles, walls, and the ground. The Ray will pass through
-- all other objects.
local target = RaycastTarget.ProjectileHit
-- Limit the ray's travel distance. We don't need to look further than
-- 500 meters.
local distance = 500
-- Perform the raycast. This returns a RaycastHit if the ray hits an
-- object on the ProjectileHit layer within 500 meters. Otherwise it
-- returns nil.
local hit = Physics.Raycast(ray, distance, target)
-- Did the ray hit an object within 500 meters?
if hit ~= nil then
-- Yes! The ray hit an object. Store its range for printing to the
-- console.
distance = hit.distance
end
-- Prints the distance to the Ravenscript console. Note that `print()`
-- accepts a string. Since `distance` is a number it must be converted
-- into a string using the `tostring()` function. This function can
-- convert most values into strings: numbers, booleans, nil, tables,
-- etc. Use `..` to concatenate two strings.
print("Distance: " .. tostring(distance))
end
The same script as above but without comments:
behaviour("Rangefinder")
function Rangefinder:Update()
local distance = 500
local target = RaycastTarget.ProjectileHit
local ray = Ray(self.transform.position, self.transform.forward)
local hit = Physics.Raycast(ray, distance, target)
if hit ~= nil then
distance = hit.distance
end
print("Distance: " .. tostring(distance))
end
Create a new text file called Rangefinder.txt
in your Unity project. Copy and paste the above code.
Create a cylinder or an empty GameObject in Unity. Attach the GameObject to a weapon. Make sure to orient the GameObject so that its Z-axis aligns with the weapon’s shooting direction. The Z-axis is indicated by the blue arrow. Attach a ScriptedBehaviour and add the Rangefinder
script as source.
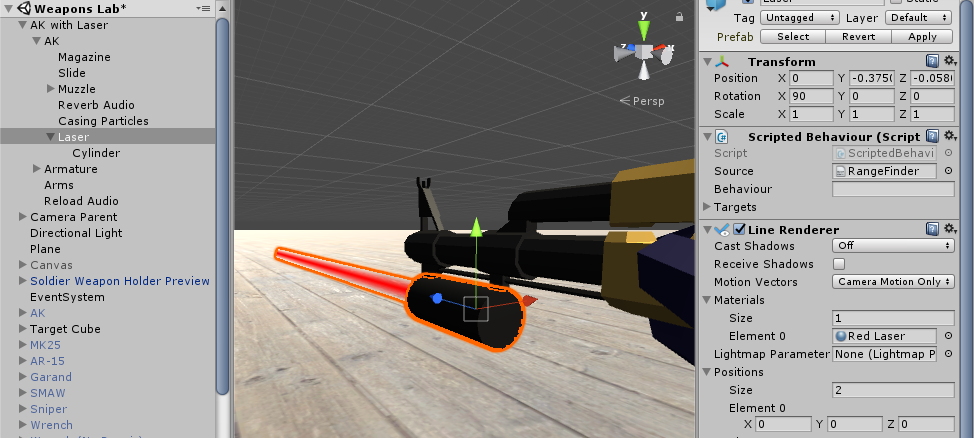