Getting started
Note
Complete the installation guide first!
What is Ravenscript?
Ravenscript is a scripting language for Ravenfield. It is part of Ravenfield’s modding tools call Ravenfield Tools Pack.
Ravenscript is based on Lua – a popular scripting language used in many different games and game engines. Familiarity with programming and Lua in particular is useful, if not required, to use Ravenscript.
Example Ravenscript code:
-- This is a comment. It is ignored by Ravenscript.
-- Try to declare all functions and variables as local. Each Ravenscript mod
-- is confined to a separate environment. Rendering name collisions with
-- other mods impossible. Nevertheless, it is still good practice.
local a = 3
local function addTwo(x)
return x + 2
end
if addTwo(a) == 5 then
print("it worked!")
end
local names = {} -- An empty table
table.insert(names, "foo")
print(names[1]) -- foo
You can experiment with Lua over at repl.it.
Behaviours
Unity uses a component based system for implementing game logic. Each component is responsible for a small part of the game such as a health pool, an interactive button, or a door. A component can also implement something large and complex such as the game’s AI. Ravenscripts borrows this approach.
In C# a component is implemented by deriving from the MonoBehaviour
class. In Ravenscript we call the behaviour()
function. This function creates and registers a Ravenscript behaviour with the given name. The behaviour is added to a Unity object through the ScriptedBehaviour
component.
Ravenscripts are stored in .txt
files and treated as TextAssets
by Unity. All Ravenscripts starts with a call to behaviour()
to create and register a class. It is recommended to use the same name for the behaviour and the .txt
file.
The ScriptedBehaviour
component accepts a Ravenscript TextAsset
. Add this component to your Unity objects to apply Ravenscript to your modded weapons, vehicles, levels, etc.
Your first script
For our first script we will limit ourselves to printing a debug message. This is not very exciting. However, it will show the necessary steps to create a simple Ravenfield mod with a single custom weapon.
Start Unity and open the Ravenscript Tools Pack.
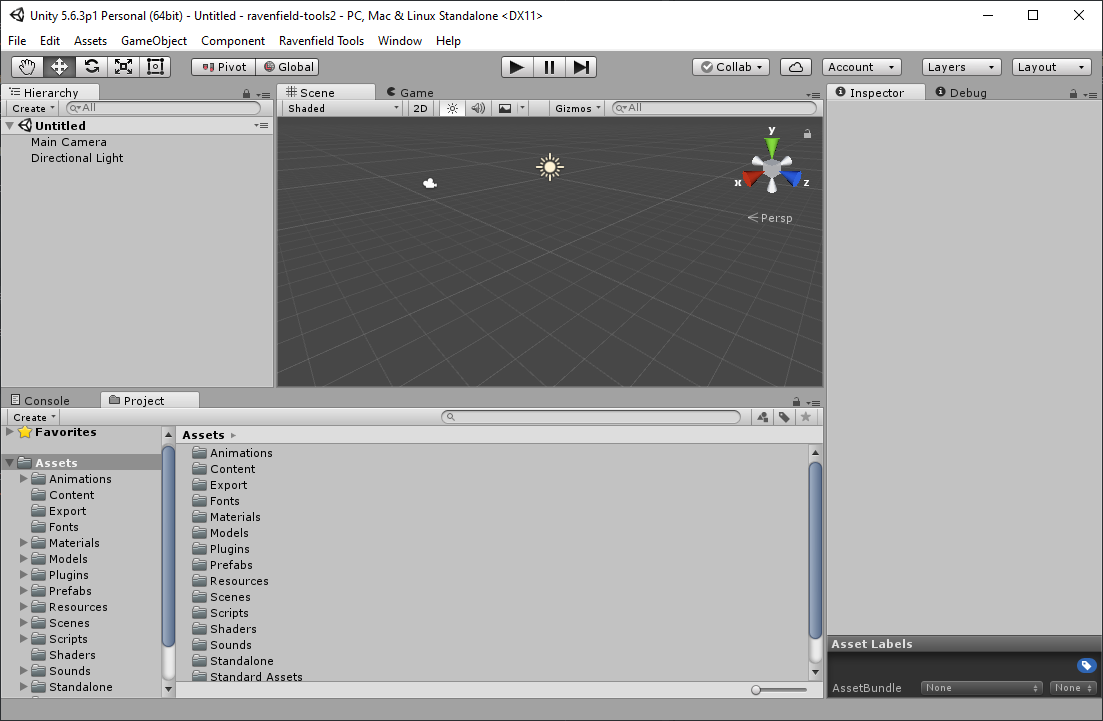
Figure: Unity with an empty scene.
Our first object is to create a new Weapon Content Mod prefab. We will attach our first Ravenscript to a Weapon then export the weapon through a content mod.
Create the content mod:
Under the
Assets
folder create a new folder calledMyMod
Find the Example Weapon Content Mod prefab in the
Assets/Content
folder.With the Example Weapon Content Mod prefab selected, press Ctrl+D to create a duplicate. Rename the duplicate to MyContent
Move the duplicated prefab to the
MyMod
folder.
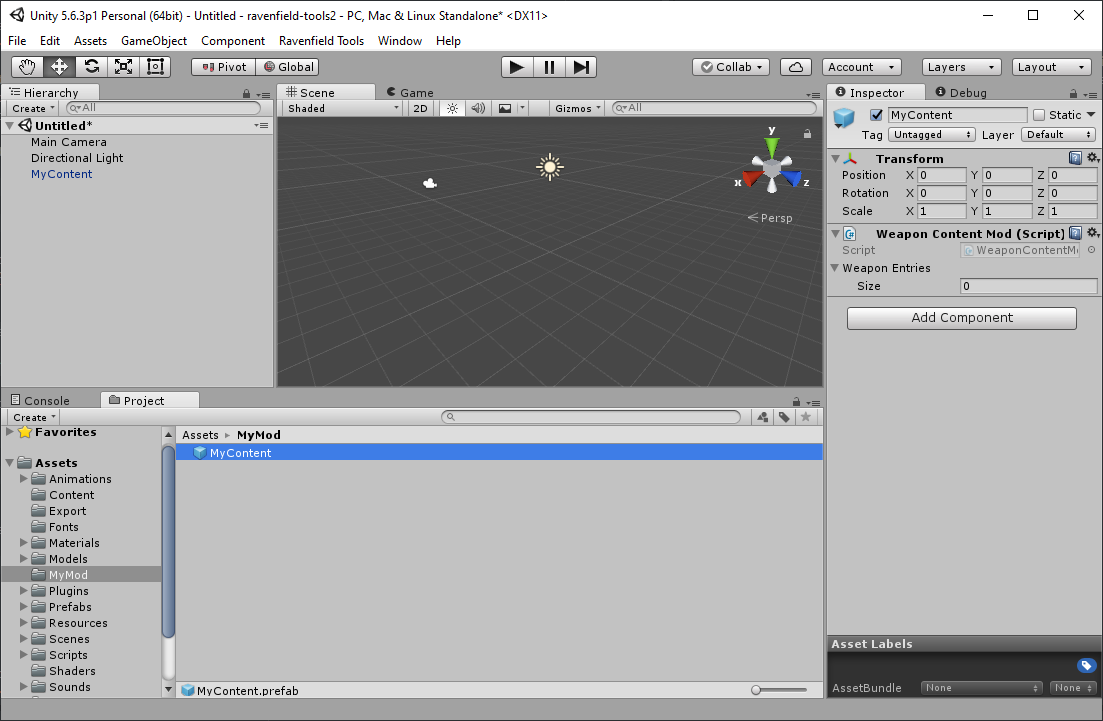
Figure: We have created a new content mode prefab named MyContent
We will attach the script to the AR 15-GL
weapon. Our content mod must therefor export the customized AR 15-GL
:
With the
MyContent
prefab selected, change the Weapon Entries Size to 1Set Name to
My AR 15-GL
Set Prefab to the
AR 15-GL
prefab located in thePrefabs/Weapons
folder
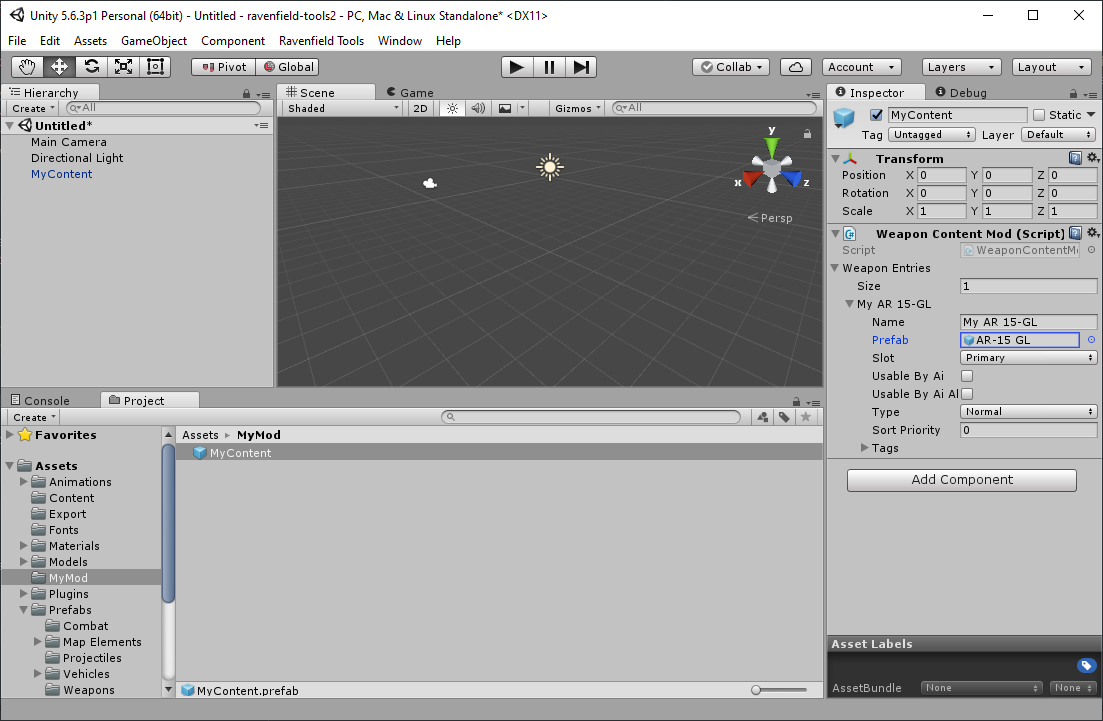
Figure: MyContent will export the AR 15-GL weapon
In the Project tab, right click next to the MyContent prefab. Select Create > Ravenscript. Name the new Ravenscript file MyBehaviour
. This creates a new Ravenscript file with the name MyBehaviour.txt
We are now ready to open Visual Studio Code (VSCode.) Start VSCode then open the Ravenscript Tools Pack folder.
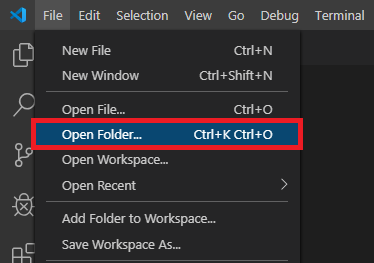
Open the MyBehaviour.txt
file under Assets/MyMod
. This file will contain our Ravenscript.
Note
The .txt
extension is mandatory in this Unity version.
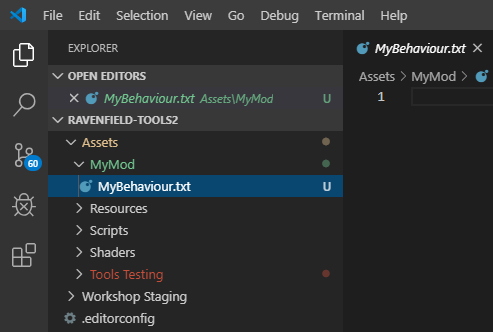
The file should already contain some code. For the purposes of this tutorial, remove any existing code and copy and paste the following Lua code into MyBehaviour.txt
:
behaviour("MyBehaviour")
function MyBehaviour:Start()
print("Hello World")
end
This script will print “Hello World” to the console. We will now attach this script to the AR 15-GL
weapon prefab.
Locate and open the Weapons Lab
scene (in the Scenes
folder.)
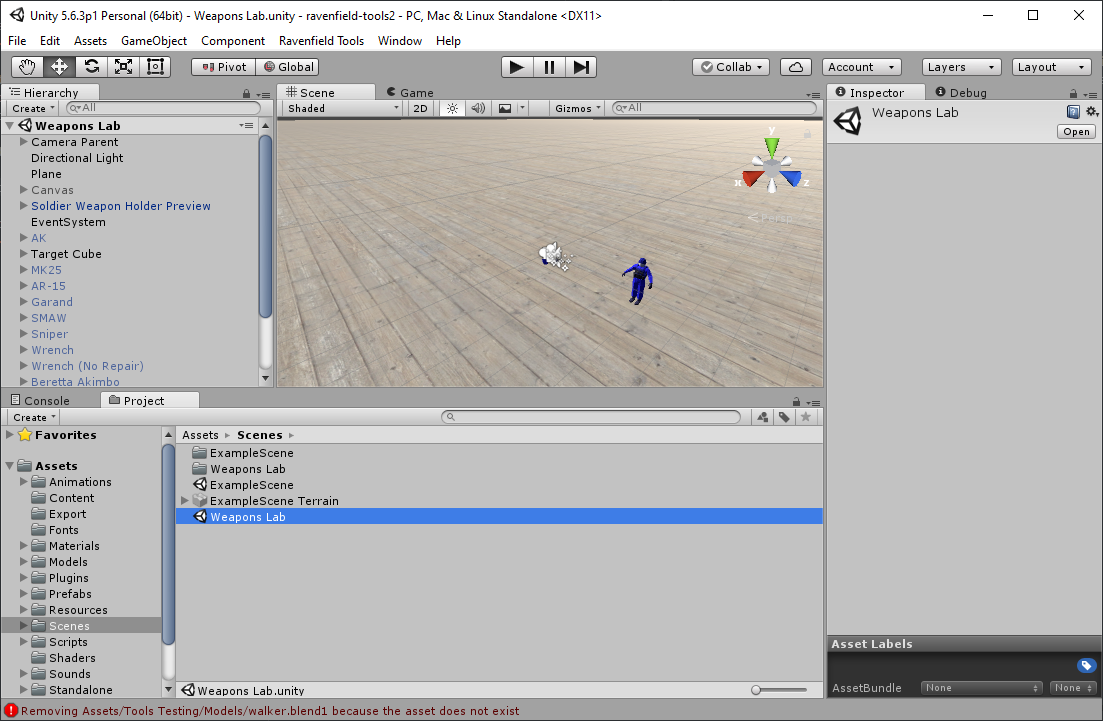
Figure: Unity with the Weapons Lab scene loaded.
Locate and select the AR 15-GL
in the scene hierarchy.
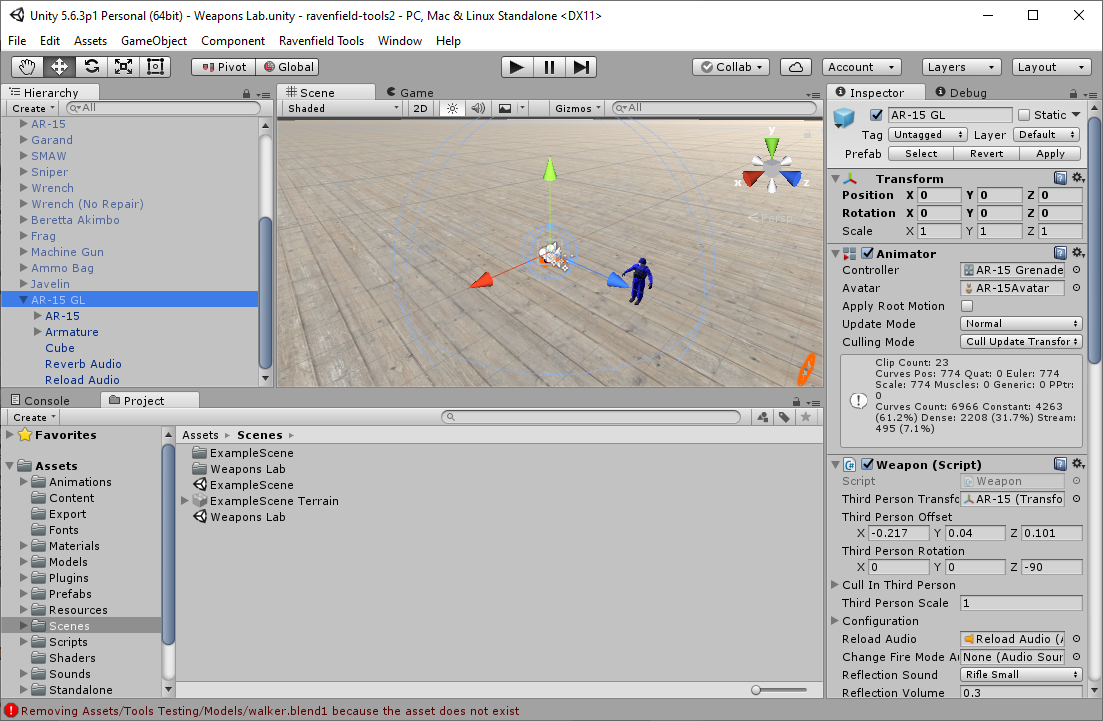
Figure: The AR 15-GL is selected from the bottom of the scene hierarchy.
With the AR 15-GL
selected, in the inspector tab, click Add Component. Add a new ScriptedBehaviour
component. Use MyBehaviour.txt
as Source.
If we leave the Behaviour field empty Ravenscript will automatically look for a behaviour with the same name as the source file. In our case it will look for a behaviour called “MyBehaviour”.
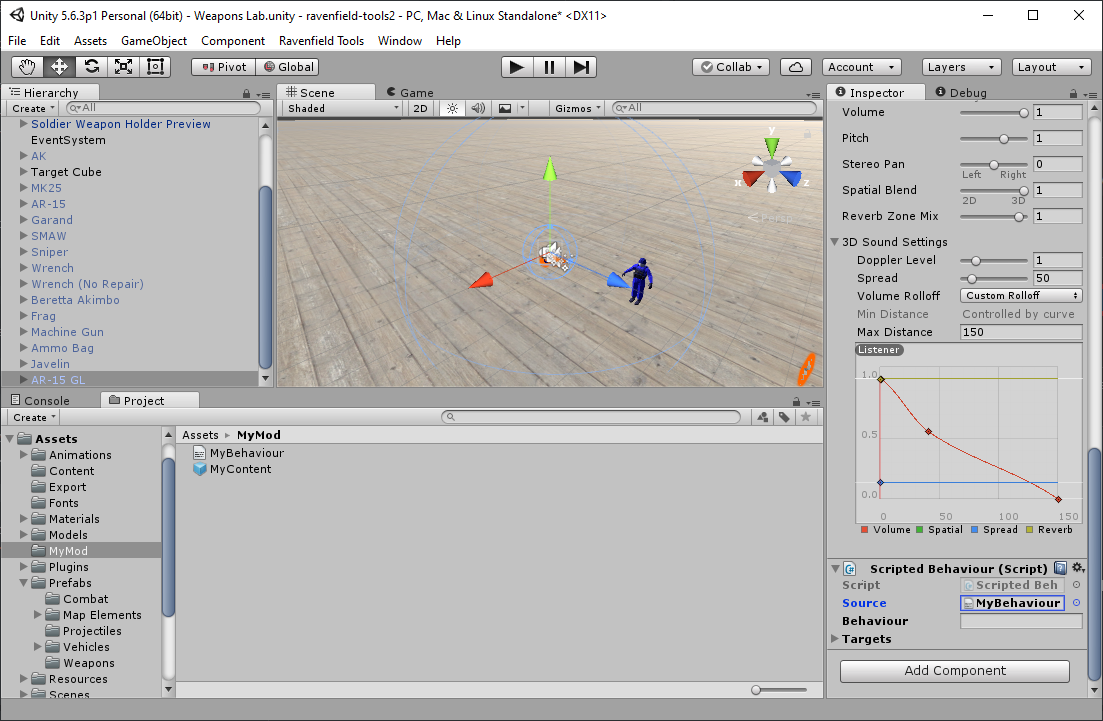
Scroll to the top of the Inspector and press the Apply button to save the changes we’ve made to the AR 15-GL
.
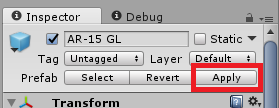
It is time to test the mod:
Select the
MyContent
prefab underAssets/MyMod
From the Unity toolbar, press Ravenfield Tools > Test Map or Content Mod
Ravenfield starts and immediately loads a test level
Select the
My AR 15-GL
rifle and press DEPLOYPress Page Up on your keyboard to open the Ravenscript Console
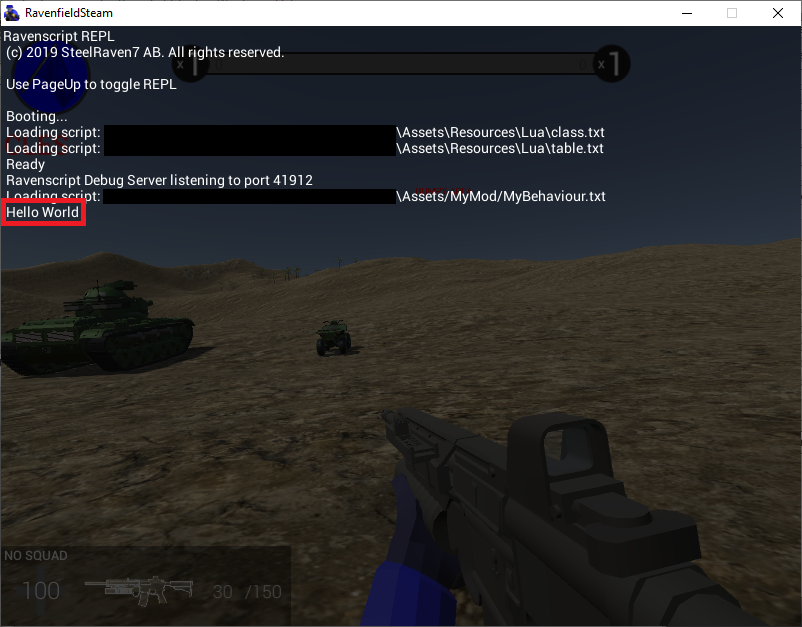
Figure: Our first script prints Hello World to the console!
Reloading scripts
When starting Ravenfield from the Ravenfield Tools Pack through Ravenfield Tools > Test Map or Content Mod the game loads your Ravenscript source files directly from your Ravenfield Tools project files. This allows you to make changes and test your script code without having to reexport the mod. Use RESTART from the game’s pause menu to reload the scene and all scripts. Or press Page Down to hot-reload all scripts (this may break if new variables are introduced). Please note that when the game is launched normally all Ravenscript code is loaded directly from the .rfc
files.
Your first bug
In the next chapter we will look at debugging your code. Maybe you have already run into a problem? Here are a few common problems and their solutions:
Console does not appear when I press Page Up
Are you using the latest version of Ravenfield?
Ravenscript support is only available on the beta branch
Did you press Apply to save the changed we made to
AR 15-GL
?Did you deploy with the
My AR 15-GL
equipped?
Unable to instantiate class ‘MyBehaviour’

Did you forget to paste the code into MyBehaviour.txt
? Open the file and have a look. Also make sure that you are using the same names everywhere. Since the source file is called MyBehaviour.txt
the Ravenscript behaviour should also be called “MyBehaviour”.